Building a Horizontally Scrolling Ticker of Images with Chakra UI
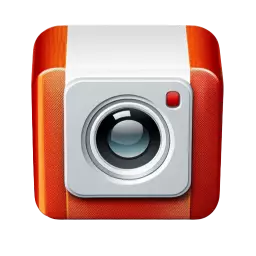
Stylized Team
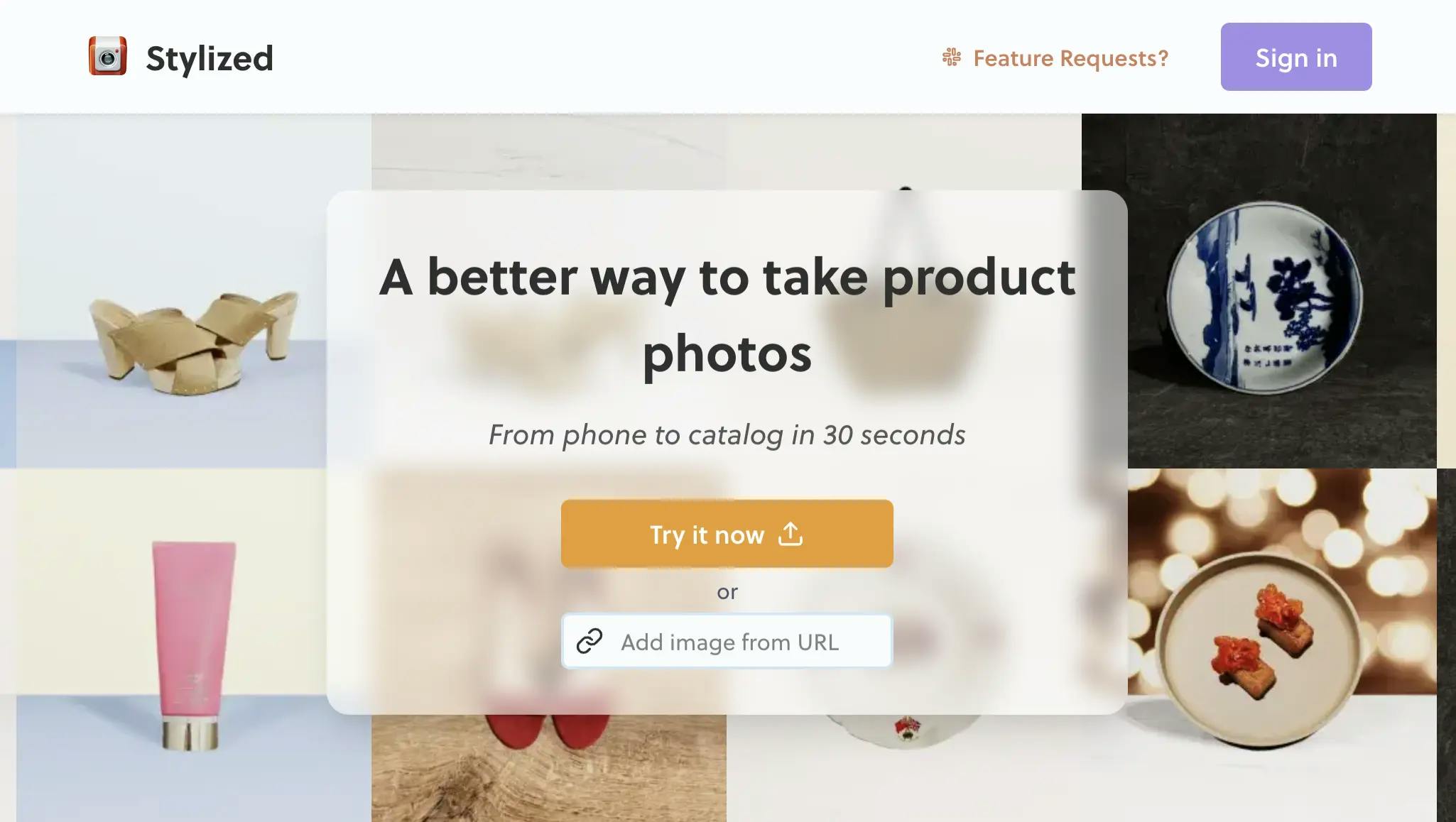
tl;dr
I recently worked on a project to create a horizontally scrolling ticker of images using the Chakra UI grid system. While the process had its challenges, I was able to turn to the Assistant for help and successfully implement the ticker.
Challenges and Lessons Learned
One of the issues I encountered was that the scrolling wasn't working correctly on mobile devices. This was a major problem because I wanted the ticker to be accessible to as many users as possible, regardless of the device they were using. Initially, I tried using a hacky solution involving setTimeout
and scrolling one image at a time, but this didn't solve the issue.
This is how my code was set up to animate the ticker:
function scrollTicker() {
ticker.scrollBy(0.5, 0);
requestAnimationFrame(scrollTicker);
}
animate(scrollTicker);
And this is the hack I thought I had to put in for mobile:
setTimeout(function() {
ticker.scrollBy(400, 0);
}, 2000);
After some further investigation, I discovered that the problem was caused by the use of half-pixel values, which are not supported on mobile. Once I increased the pixel value to a whole number, the scrolling started working correctly on mobile devices. This was a valuable lesson for me in the importance of testing on different devices and being aware of device-specific limitations.
Another challenge I faced was finding a way to add new nodes to the grid without disrupting the flow of the ticker. Whenever I tried to remove a node, it caused everything to reshuffle, which disrupted the continuity of the ticker. This was frustrating because I wanted the ticker to flow smoothly, without any interruptions or disruptions. I tried various approaches to solve this problem, but ultimately I was not able to find a satisfactory solution. This was a lesson in the importance of thoroughly testing and debugging code to ensure that it works as intended.
Optimization and Final Result
To optimize the ticker and ensure that it performed well, I wrote a script to convert the images from the PNG format to the more efficient WebP format. I also used the script to resize the images so that 40 of them would fit within a size limit of 300kb. This was an important step because it helped reduce the load time of the ticker, making it more enjoyable for users to interact with. The script was a valuable tool that helped me optimize the ticker and ensure that it was performing at its best.
const fs = require('fs');
const webp = require('webp-converter');
const inputDir = '/Users/kumquatexpress/Downloads/pngstoconvert';
const outputDir = '/Users/kumquatexpress/Downloads/webpout';
fs.readdir(inputDir, (err, files) => {
if (err) {
console.error(err);
return;
}
files.forEach((file, idx) => {
const inputPath = `${inputDir}/${file}`;
const outputPath = `${outputDir}/${idx}.webp`;
console.log("inputPath", inputPath)
webp.cwebp(inputPath, outputPath, '-q 80')
});
});
The end result of my work on the ticker was a much more professional hero image. I was pleased with the final product and felt that it represented a significant improvement over the previous version. I was also excited to release the new hero image as part of a revamp of our landing page. The revamp represented a major overhaul of the page, with a focus on improving the user experience and making the site more visually appealing. I was proud to have contributed to this effort and was excited to see the new landing page go live.
Closing Thoughts
The end result of my work on the ticker was a much more professional hero image. I was pleased with the final product and felt that it represented a significant improvement over the previous version. I was also excited to release the new hero image as part of a revamp of our landing page. The revamp represented a major overhaul of the page, with a focus on improving the user experience and making the site more visually appealing. I was proud to have contributed to this effort and was excited to see the new landing page go live.
If you enjoyed reading about my experiences and lessons learned while building a horizontally scrolling ticker of images with Chakra UI, be sure to check out what we’re building at stylized.ai! We regularly post articles on a variety of topics related to design and development. Thanks for reading!